4 Create a simple image#
Objectives#
synthesize an RGB image (and know, for example, that yellow = green + red)
manipulate the value / color correspondence
use the
:
operator
Image synthesis#
As usual, do not forget the modules:
import numpy as np
import matplotlib.pyplot as plt
The image is composed of blocks of homogeneous color with size 20 × 20 pixels, so as to form an image of 40 × 80 pixels.
Also, it contains three bands as it is an RGB image.
Therefore we create an array f
of size 40 × 80 × 3:
f = np.zeros((40,80,3))
We use Additive color to create the colors. Thus, yellow is obtained by combining green and red.
f[ 0:20 , : , 0 ] = 1 # Red band = band 0 (top row: pixels 0 to 19)
f[ : , 0:40 , 1 ] = 1 # Green band = band 1 (the two columns on the left)
f[ : , 20:60 , 2 ] = 1 # Blue band = band 2 (the two columns at the center)
The bands can be displayed separately:
plt.figure(figsize=(15,7))
plt.subplot(1,3,1)
plt.imshow(f[:,:,0], cmap="gray")
plt.title('Red band')
plt.subplot(1,3,2)
plt.imshow(f[:,:,1], cmap="gray")
plt.title('Green band')
plt.subplot(1,3,3)
plt.imshow(f[:,:,2], cmap="gray")
plt.title('Blue band')
plt.show()

And finally the color image:
plt.imshow(f)
plt.show()
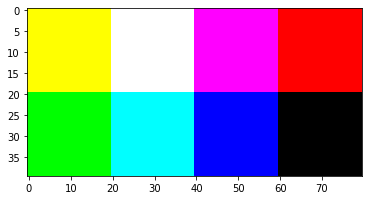